Which Go Web Backend Framework Is Right for Your Next Project? A Detailed Analysis of Features and Performance
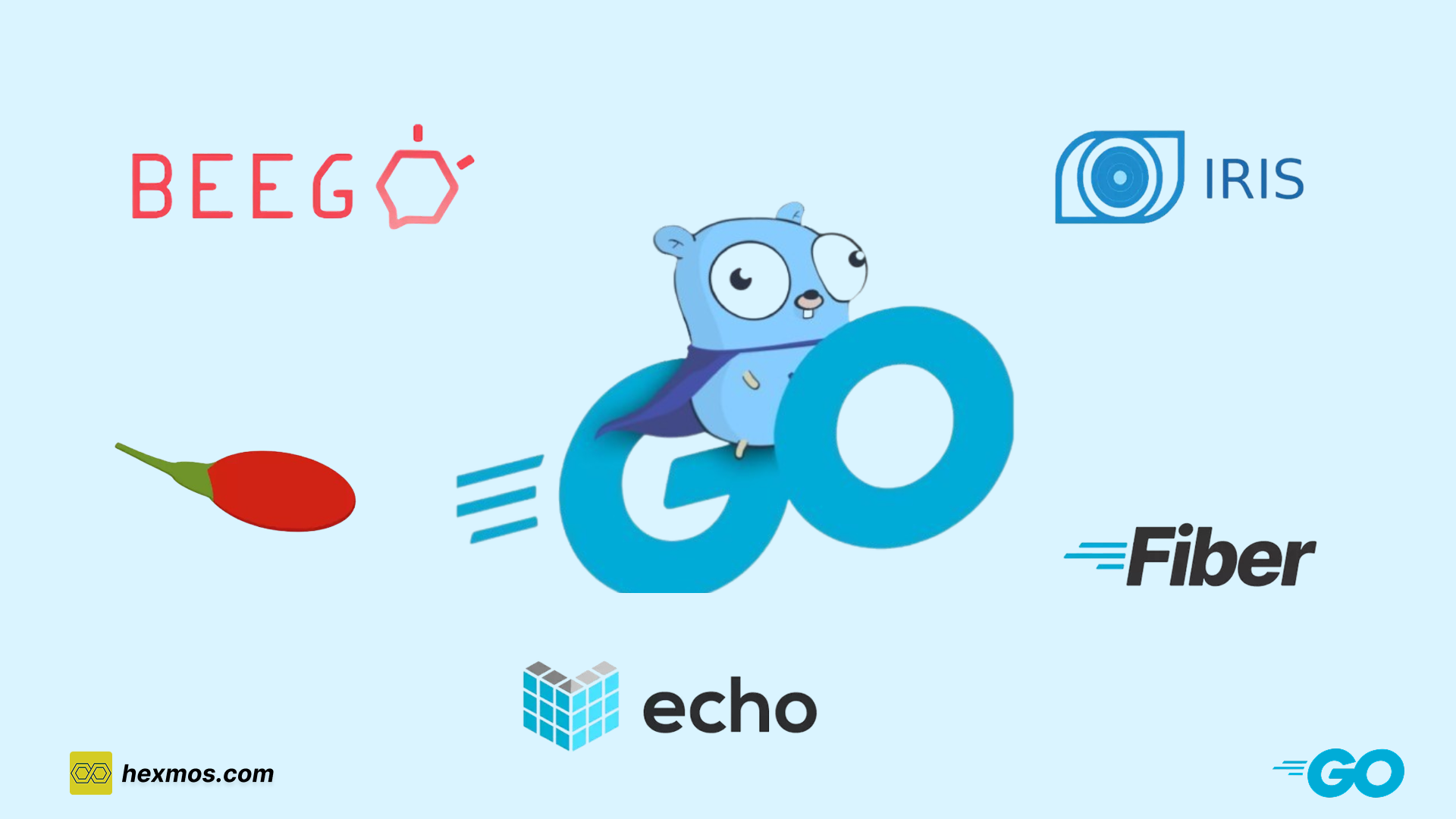
If you're beginning backend development with Go and wondering which framework to choose, in this blog we'll explore some popular frameworks in the Go ecosystem, outlining their key features and performance metrics with practical examples to help you make an informed choice.
Go, created by Google, has become a favorite in the backend world thanks to its simplicity, speed, and built-in concurrency through goroutines and channels. Its compiled nature allows for highly performant applications that often outperform interpreted languages, making it an excellent choice for robust backend systems.
While Go’s built-in net/http
package is powerful, leveraging a web framework can streamline development. Frameworks provide essential tools for routing, data handling, and middleware integration—allowing you to concentrate on designing unique application logic rather than reinventing foundational components. Think of it as using a pre-assembled chassis for a car, offering a reliable platform on which to build advanced features.
This guide offers a detailed technical analysis of framework options in the Go ecosystem, examining performance, scalability, and integration ease to ensure you select the right tool for your next project.
Framework Deep Dive: Features
With a clear overview of the top frameworks, let's dive deeper into each one. In the following sections, we'll examine the unique features and core functionalities of each framework, providing insights into what makes them stand out in the Go ecosystem.
Beego
Overview:
Beego is often characterized as a comprehensive, or "full-stack", framework, drawing parallels to the well-established Django framework in the Python ecosystem. It is designed to facilitate the rapid development of enterprise-grade applications and RESTful APIs by providing a wealth of built-in features.
Architecture & Design
The framework embraces the Model-View-Controller (MVC) architectural pattern, promoting a clear separation between the application's data (Model), its presentation (View), and the logic handling user input (Controller). This structure is particularly helpful for beginners, offering a clear roadmap for organizing code.
Database Integration
Additionally, Beego includes a robust Object-Relational Mapper (ORM) that simplifies database interactions. Instead of writing complex SQL queries, developers can manipulate data using Go objects while the ORM handles the translation to and from the database. It supports popular database systems such as MySQL, PostgreSQL, and SQLite.
Batteries-Included Philosophy
Beego follows a "batteries included" philosophy, offering built-in modules for managing user sessions, logging application activity, caching data for faster retrieval, handling configuration settings, and even supporting internationalization (i18n). This comprehensive feature set reduces the need to search for and integrate numerous external libraries.
Automation with Bee Tool
Moreover, Beego provides a powerful command-line tool called "Bee Tool" that automates common development tasks like creating new projects, generating code, building the application, running tests, and deploying it. This automation significantly streamlines the workflow, especially for those new to the development process.
Ideal For
Beego is an excellent choice for developers and teams looking to build robust, enterprise-grade applications quickly. It is especially suitable for those who prefer an all-in-one solution that minimizes the need for third-party libraries. With its comprehensive feature set—including built-in ORM, session management, caching, and internationalization support—Beego offers a solid foundation for developing complex applications and RESTful APIs. Beginners will appreciate the clear MVC structure and the automation provided by the Bee Tool, which simplifies many repetitive development tasks.
"Hello World" Example:
package main
import "github.com/beego/beego/v2/server/web"
type MainController struct {
web.Controller
}
func (c *MainController) Get() {
c.Ctx.WriteString("Hello, World from Beego!")
}
func main() {
web.Router("/", &MainController{})
web.Run()
}
- Import Package: We import the
web
package from the Beego framework. - Define Controller: We define a controller
MainController
that embedsweb.Controller
. - Handle GET Request: The
Get()
method handles HTTP GET requests to the root path (/
). - Write Response:
c.Ctx.WriteString()
writes the "Hello, World!" message to the response. - Register Route: In
main()
, we register the router, mapping the root path to ourMainController
. - Start Server:
web.Run()
starts the Beego application.
To run this:
Make sure you have Beego installed by running:
go install github.com/beego/beego/v2/server/web
Save the code as main.go
and run go run main.go
. Then, navigate to http://localhost:8080 in your browser.
Echo
Overview:
Echo is known as a high-performance, extensible, and minimalist framework with a focus on building microservices, APIs, and JSON web services. It is designed to maximize speed and developer productivity without the burden of unnecessary features.
Performance & Design
At its core, Echo features a highly optimized HTTP router that efficiently directs incoming requests to the appropriate handlers, minimizing dynamic memory allocation. Its minimalist design provides only the essential building blocks for web development, making it easier for beginners to grasp fundamental concepts.
API Building & Data Binding
Echo excels at building RESTful APIs by offering features like automatic data binding—which converts incoming request data (in formats like JSON, XML, or form data) into Go data structures—and data rendering for sending responses in various formats.
Middleware Support
A key strength of Echo is its flexible middleware system, which allows for seamless integration of functionalities such as logging, user authentication, and CORS management. Middleware can be applied globally, to specific route groups, or individually.
Security & TLS
One particularly helpful feature for beginners is Echo's ability to automatically handle TLS certificate installation using Let's Encrypt, simplifying the process of securing your web applications with HTTPS.
Ideal For
Echo is ideal for developers seeking a lightweight yet powerful framework for building RESTful APIs and microservices. Its streamlined design, high performance, and easy middleware integration make it an excellent choice for projects where security and speed are paramount.
"Hello World" Example:
package main
import (
"net/http"
"github.com/labstack/echo/v4"
)
func main() {
e := echo.New()
e.GET("/", func(c echo.Context) error {
return c.String(http.StatusOK, "Hello, World from Echo!")
})
e.Logger.Fatal(e.Start(":1323"))
}
- Import Package: We import the
echo
package. - Create Instance: A new Echo instance is created using
echo.New()
. - Define Route: A route for the root path (
/
) is defined usinge.GET()
. - Handle Request: The handler sends a "Hello, World!" response with HTTP status 200 (StatusOK).
- Start Server:
e.Start(":1323")
starts the Echo server on port 1323.
To run this:
Install Echo by running:
go get github.com/labstack/echo/v4
Save the code as main.go
and run go run main.go
. Access http://localhost:1323 in your browser.
Fiber
Overview:
Fiber draws direct inspiration from Express.js, a widely used web framework in the Node.js ecosystem. It is built on top of Fasthttp, known for its exceptional speed and low memory usage.
Routing & Performance
Fiber offers a robust and intuitive routing system that allows developers to easily define endpoints and handle various HTTP methods (e.g., GET, POST, PUT, DELETE). Its foundation on Fasthttp makes it a high-performance option for demanding applications.
Middleware & Templating
Fiber provides extensive middleware support for tasks such as logging, authentication, and data validation. It also supports various template engines for dynamic HTML content and includes built-in support for WebSockets for real-time communication.
Traffic Management
For managing traffic, Fiber offers middleware for rate limiting, ensuring your application remains responsive under heavy loads.
Ideal For
Fiber is perfect for developers coming from a JavaScript background or anyone who appreciates an Express.js-like syntax. Its intuitive API and high-performance routing make it a strong choice for building fast, scalable applications.
"Hello World" Example:
package main
import "github.com/gofiber/fiber/v2"
func main() {
app := fiber.New()
app.Get("/", func(c *fiber.Ctx) error {
return c.SendString("Hello, World from Fiber!")
})
app.Listen(":3000")
}
- Import Package: We import the
fiber
package. - Create Instance: A new Fiber application instance is created using
fiber.New()
. - Define Route: A route for the root path (
/
) is defined usingapp.Get()
. - Send Response: The handler sends a "Hello, World!" string response.
- Start Server:
app.Listen(":3000")
starts the Fiber server on port 3000.
To run this:
Install Fiber by running:
go get github.com/gofiber/fiber/v2
Save the code as main.go
and run go run main.go
. Open http://localhost:3000 in your browser.
Iris
Overview:
Iris is a fast, modern, and easy-to-learn HTTP/2 Go web framework that offers a rich set of features, including support for the Model-View-Controller (MVC) architectural pattern and extensive middleware capabilities.
Advanced Features & Performance
Iris positions itself as the fastest HTTP/2 framework, offering advanced features like HTTP/2 Push to boost web application performance. It also supports API versioning, WebSockets, and gRPC for building high-performance services.
Flexible Routing & Middleware
Iris boasts a powerful router for handling incoming requests, along with features like response compression, support for multiple view engines, and even localization (i18n) capabilities. Its extensive middleware options facilitate the integration of additional functionalities such as logging and authentication.
Ideal For
Iris is well-suited for developers building complex, large-scale applications that require advanced performance features and robust support for real-time communication. Although its rich feature set may present a steeper learning curve, Iris offers tremendous control and scalability for demanding projects.
"Hello World" Example:
package main
import "github.com/kataras/iris/v12"
func main() {
app := iris.New()
app.Get("/", func(ctx iris.Context) {
ctx.WriteString("Hello, World from Iris!")
})
app.Listen(":8080")
}
- Import Package: We import the
iris
package. - Create Instance: A new Iris application instance is created using
iris.New()
. - Define Route: A route for the root path (
/
) is defined usingapp.Get()
. - Send Response: The handler writes "Hello, World!" to the response.
- Start Server:
app.Listen(":8080")
starts the Iris server on port 8080.
To run this:
Install Iris by running:
go get github.com/kataras/iris/v12
Save the code as main.go
and run go run main.go
. Access http://localhost:8080 in your browser.
Goji
Overview:
Goji distinguishes itself as a lightweight and minimalistic HTTP request multiplexer that values composability and simplicity. It is built directly on top of Go's standard net/http
package, offering a fundamental and less opinionated approach to web development.
Core Approach & Flexibility
Goji emphasizes a close alignment with the standard net/http
package, enabling seamless use of standard Go HTTP handlers and middleware components. Its routing mechanism supports both Sinatra-style URL patterns (e.g., /users/:id
) and regular expressions, providing flexibility in endpoint definition.
Ideal For
Goji is ideal for developers who prefer a minimalistic and transparent approach to building web applications. It is best suited for those who want to understand the inner workings of Go’s HTTP handling and appreciate the flexibility of composing their own middleware. Goji’s lightweight nature and direct use of the standard library make it an excellent choice for small-scale projects and microservices where simplicity and performance are key.
"Hello World" Example:
package main
import (
"fmt"
"net/http"
"github.com/goji/goji/v3/pat"
"github.com/goji/goji/v3/web"
)
func hello(c web.C, w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, World from Goji!")
}
func main() {
mux := web.New()
mux.Get(pat.Get("/"), hello)
http.ListenAndServe(":8000", mux)
}
- Import Packages: Necessary packages such as
goji/web
andgoji/pat
are imported. - Define Handler: A handler function
hello
is defined, which takes aweb.C
,http.ResponseWriter
, andhttp.Request
as arguments. - Send Response: Inside the handler,
fmt.Fprintf
writes "Hello, World!" to the response. - Create Multiplexer: A new Goji multiplexer is created using
web.New()
. - Register Route: A route for the root path (
/
) is registered usingmux.Get()
. - Start Server:
http.ListenAndServe(":8000", mux)
starts the HTTP server on port 8000.
To run this:
Install Goji by running:
go get github.com/goji/goji/v3
Save the code as main.go
and run go run main.go
. Navigate to http://localhost:8000 in your browser.
Performance Under Pressure: Load Capacity Benchmarks
When choosing a backend framework, understanding its performance is crucial. Load capacity measures how many concurrent requests a server can handle without performance drops. Benchmarks typically focus on requests per second (RPS) and latency, providing insights into a framework's scalability.
Key Considerations:
- Variability: Results can differ based on test setup, hardware, and workload.
- Multiple Sources: Always cross-reference benchmarks for a complete picture.
- Real-World Impact: Production performance also depends on database efficiency, business logic, and network conditions.
Summary Table:
Framework | General Performance Ranking | Key Performance Strengths (based on benchmarks) | Notable Benchmark Trends |
---|---|---|---|
Beego | Fast | Good performance under various loads | Consistently delivers reliable performance across diverse workloads, showcasing its strength in complex, real-world scenarios. |
Echo | Very Fast | Consistently ranks high in various benchmarks, including "hello world" scenarios | Strong performance and efficient resource utilization. |
Fiber | Very Fast | Frequently achieves top performance in benchmarks, leveraging Fasthttp's speed | Performance in basic tests can be competitive but not always the absolute fastest. |
Iris | Fast | Claims to be the fastest HTTP/2 framework; performs well in some "hello world" benchmarks | Limited direct benchmark data available in the provided snippets. |
Goji | Fast | Lightweight design contributes to good performance, as shown | Focus on efficiency and minimal overhead. |
Note: This performance data is taken from the smallnest/go-web-framework-benchmark repository.
This concise overview should help you decide which framework best meets your anticipated load and application needs.
Conclusion: Your First Step into the Go Backend World
As you stand at the threshold of your Go backend development journey, you now have a clearer picture of five popular frameworks that can serve as your foundation: Beego, with its comprehensive feature set reminiscent of Django; Echo, known for its performant and minimalist API; Fiber, the fast and familiar option for those coming from Express.js; Iris, the feature-rich framework boasting HTTP/2 prowess; and Goji, the lightweight and composable choice for a more fundamental approach.
The most important step now is to take action. Visit the official documentation for the frameworks that have piqued your interest. Try building a simple "hello world" application or a small project to get a hands-on feel for how each one works. Remember that the knowledge and skills you acquire with one framework will undoubtedly be beneficial as you explore others in the future. Your initial choice is just the first step into the exciting world of Go backend development. So, pick a framework that resonates with you, start building, and enjoy the journey!
I hope this article helped you to learn about frameworks and their advantages. Thanks for the read. If you need API documentation for any of these frameworks, feel free to try out LiveAPI — we would love to hear your experiences.
LiveAPI: Interactive API Docs that Convert
Static API docs often lose the customer's attention before they try your APIs. With LiveAPI, developers can instantly try your APIs right from the browser, capturing their attention within the first 30 seconds.