Unlocking API Discovery: How We Nail Accuracy in LiveAPI
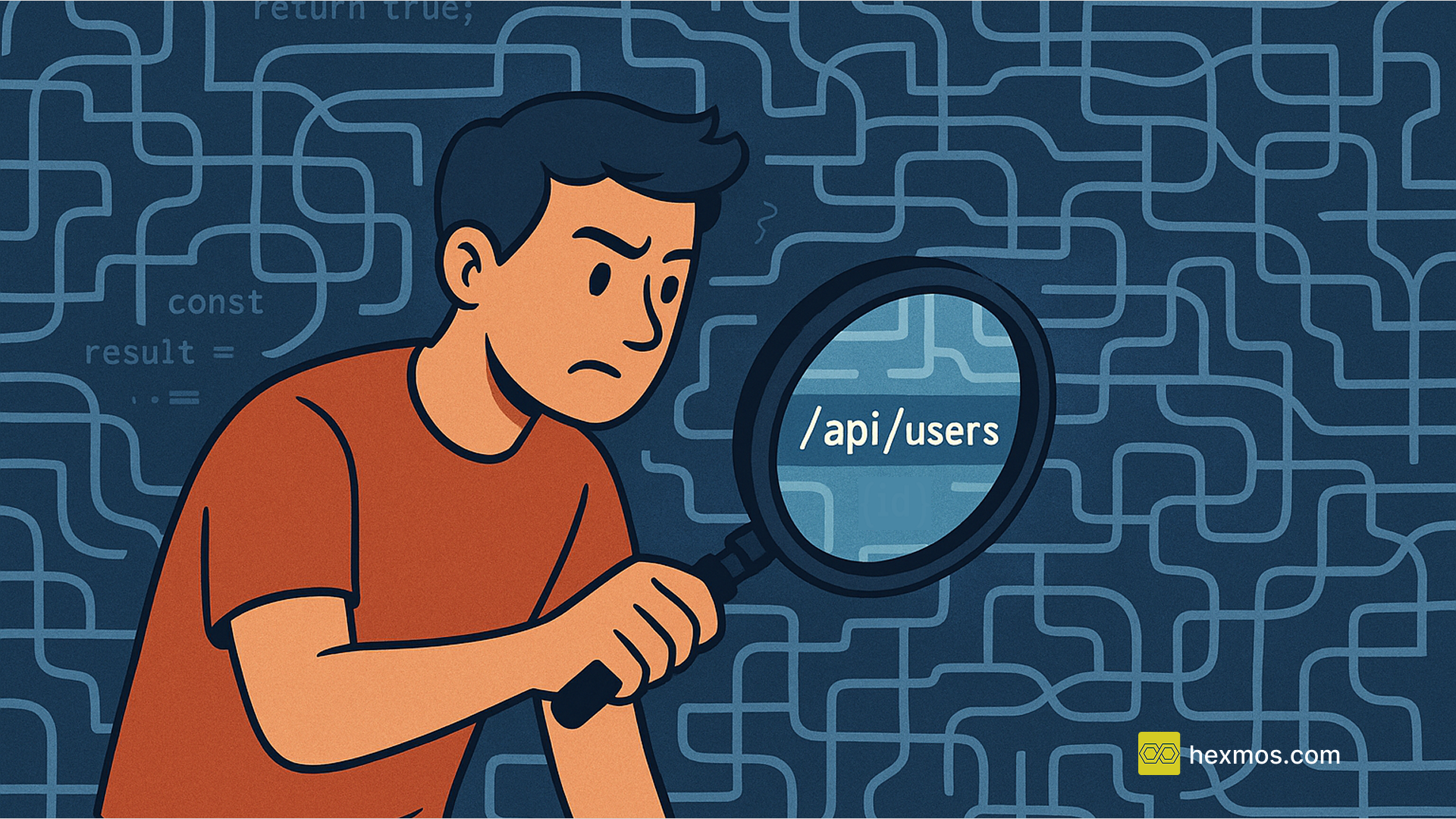
API search is a pain point for developers in large organizations. With sprawling codebases, hundreds of services, and thousands of APIs, finding the right endpoint can feel like searching for a needle in a haystack.
At LiveAPI, we’re tackling this head-on by building a system that crawls code, indexes APIs, and delivers accurate search results. In this post, I’ll dive into how we think about accuracy in LiveAPI, breaking down the challenges, our approach, and the metrics that keep us honest.
This isn’t just about slapping a search bar on a codebase. It’s about making sure developers can find what they need—fast—without pinging half the company on Slack or digging through tribal knowledge. Let’s explore how we’re making that happen.
Why API Search Is a Big Deal
In a large organization, APIs are the glue that holds microservices together. Teams build and expose APIs for others to use, but as the company grows, so does the number of APIs. Think hundreds of services and thousands of endpoints.
Without a robust search system, developers waste hours—or even days—hunting for the right API, its entry point, or its documentation. This lost productivity is a silent killer for engineering teams.
The problem boils down to this: how do you find an API in a massive, multi-language, multi-framework codebase? At LiveAPI, we’re solving this by crawling codebases to identify API entry points and making them searchable. The goal? Reduce reliance on tribal knowledge and let developers focus on building, not searching.
Key takeaway: Inefficient API search leads to lost productivity and frustration. A good search system is critical for scaling development in large organizations.
The Two Pillars of API Search: Indexing and Lookup
Our approach to API search rests on two core components: indexing and lookup. Indexing is about discovering and cataloging APIs across a codebase. Lookup is about searching that index to return relevant results. Both need to be accurate to deliver a great experience.
- Indexing: We scan codebases to detect API definitions, regardless of the language or framework. This creates a structured index of APIs, including their endpoints, methods, file paths, and repository details.
- Lookup: Once we have the index, we use search algorithms to match user queries to the right APIs, allowing filtering by endpoint, method, project name, or file path.
If either piece fails—say, the index misses APIs or the search returns irrelevant results—the whole system falls apart. Accuracy in both is non-negotiable.
Key takeaway: Indexing builds the foundation, and lookup delivers the results. Both must be accurate for API search to work.
Indexing: Catching Every API, Every Time
Indexing is the backbone of LiveAPI. It’s about detecting APIs across diverse codebases—think Python, Java, Go, and more, each using different frameworks like Flask, Spring, or Express.
The challenge is huge: we support over 10 languages and 30 frameworks, and each has its own way of defining APIs. Missing even one endpoint can break the search experience.
Here’s how we do it:
- Automated Detection: We scan code to identify API entry points, like HTTP methods (GET, POST, etc.) and their associated paths.
- Structured Output: For each API, we store details like the endpoint, method, file path, and repository name in a structured index.
For example, imagine a Python Flask app with an endpoint:
from flask import Flask
app = Flask(__name__)
@app.route('/users/<id>', methods=['GET'])
def get_user(id):
return {"user_id": id}
if __name__ == '__main__':
app.run(debug=True)
# Output: Index entry created for GET /users/<id> in file app.py, repo user-service
This code gets scanned, and we extract the endpoint /users/<id>
, method GET
, and its location. The result is a structured index entry that’s ready for search.
Key takeaway: Accurate indexing requires robust detection across languages and frameworks. Our system handles this diversity to ensure no API is left behind.
Measuring Indexing Accuracy: Recall and Precision
How do we know our indexing is accurate? We rely on two metrics: recall and precision. These are the gold standards for evaluating how well we’re detecting APIs.
- Recall: The percentage of actual APIs we correctly identify. If a codebase has 10 APIs and we find 8, but only 5 are correct, our recall is 5/10 = 50%.
- Precision: The percentage of detected APIs that are correct. Using the same example, if we report 8 APIs but only 5 are correct, our precision is 5/8 = 62.5%.
Here’s a table to illustrate:
Metric | Formula | Example (10 APIs, 8 reported, 5 correct) |
---|---|---|
Recall | Correct / Total APIs | 5 / 10 = 50% |
Precision | Correct / Reported | 5 / 8 = 62.5% |
Our current recall is around 75%, and we’re targeting 90-95%. Precision is trickier, sitting at 60-70%, but we’re improving both by testing against a dataset of 80-90 projects. Every code change triggers automated tests to track these metrics, ensuring we don’t regress.
Key takeaway: Recall and precision measure how well we index APIs. High scores in both mean a reliable index for search.
Search Quality: Finding What You Need
Once we have a solid index, the next step is search quality. This is about taking a user’s query—like “GET /users” or “auth service endpoint”—and returning the most relevant results. We use standard algorithms (like naive search) to match queries against the index, supporting parameters like:
- Endpoint (e.g.,
/users/<id>
) - HTTP method (e.g.,
GET
,POST
) - Project or repository name
- File path
For example, a developer might query:
GET /users
Our system searches the index and returns results like:
[
{
"endpoint": "/users/<id>",
"method": "GET",
"file": "app.py",
"line": 10,
"repo": "user-service",
"path": "src/app.py"
}
]
# Output: Returns matching APIs with their details
We also allow filtering to narrow results, like specifying the repo or method. This flexibility is key to cutting through the noise in large codebases.
Key takeaway: Search quality depends on matching queries to the index accurately and providing flexible filters to refine results.
Handling Multi-Language Codebases
Large organizations don’t stick to one language or framework. A single codebase might have APIs in Python (Flask), Java (Spring), and JavaScript (Express). Our system is built to handle this diversity, ensuring consistent indexing and search across languages.
For example, here’s how we index a Java Spring endpoint:
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
return new User(id, "John Doe");
}
}
# Output: Index entry created for GET /api/users/{id} in file UserController.java, repo user-service
We parse the @GetMapping
annotation to extract the endpoint and method, just like we parse Flask’s @app.route
in Python. This multi-language support is critical for organizations with diverse tech stacks.
Key takeaway: Supporting multiple languages and frameworks ensures our system works for any codebase, no matter how varied.
Learn more about Spring annotations: Spring Documentation
Improving Accuracy: Our Testing Approach
Accuracy doesn’t happen by accident. We’ve built a rigorous testing pipeline to keep our indexing and search quality high. Every change to LiveAPI is tested against a dataset of 80-90 real-world projects, covering different languages, frameworks, and API styles. We track recall and precision metrics for each run, plotting them on graphs to monitor progress.
For example, if we tweak our Python parser to better detect Flask endpoints, we run it against our dataset and check:
- Did recall improve (are we finding more APIs)?
- Did precision hold steady (are we avoiding false positives)?
This iterative approach has pushed our recall from 60% to 75% over time, and we’re gunning for 90-95%. Precision is improving too, though it’s a tougher nut to crack due to framework quirks and edge cases.
Key takeaway: Continuous testing against diverse projects ensures our accuracy keeps improving, with clear metrics to guide us.
What’s Next for LiveAPI Accuracy
So, where do we go from here? Our current recall of 75% is solid, but we’re not stopping until we hit 90-95%. Precision needs work too—getting above 80% is the goal. We’re also exploring ways to enhance search quality, like adding smarter ranking algorithms to prioritize the most relevant results.
Another focus is improving support for niche frameworks and languages to make our system even more universal.
For developers, this means less time hunting for APIs and more time building. For organizations, it means less reliance on tribal knowledge and faster onboarding for new engineers. LiveAPI is about making API discovery seamless, and accuracy is the key to that promise.
If you’re dealing with API sprawl in your organization, tools like LiveAPI can be a game-changer. Check out our progress at our website and let us know how you’re tackling API search in your own projects.
Key takeaway: We’re pushing for higher recall and precision while expanding language support, all to make API discovery effortless for developers.
This post should give you a clear picture of how we’re tackling accuracy in LiveAPI. By focusing on robust indexing, precise search, and continuous testing, we’re building a tool that saves developers time and frustration. Got thoughts or questions? Drop them in the comments—I’d love to hear how you’re solving the API search problem!
LiveAPI helps you get all your backend APIs documented in a few minutes
With LiveAPI, you can quickly generate interactive API documentation that allows users to search and execute APIs directly from the browser.
If you’re tired of manually creating docs for your APIs, this tool might just make your life easier.