Auto-Formatting with Prettier, Husky & lint-staged on Every Git Commit
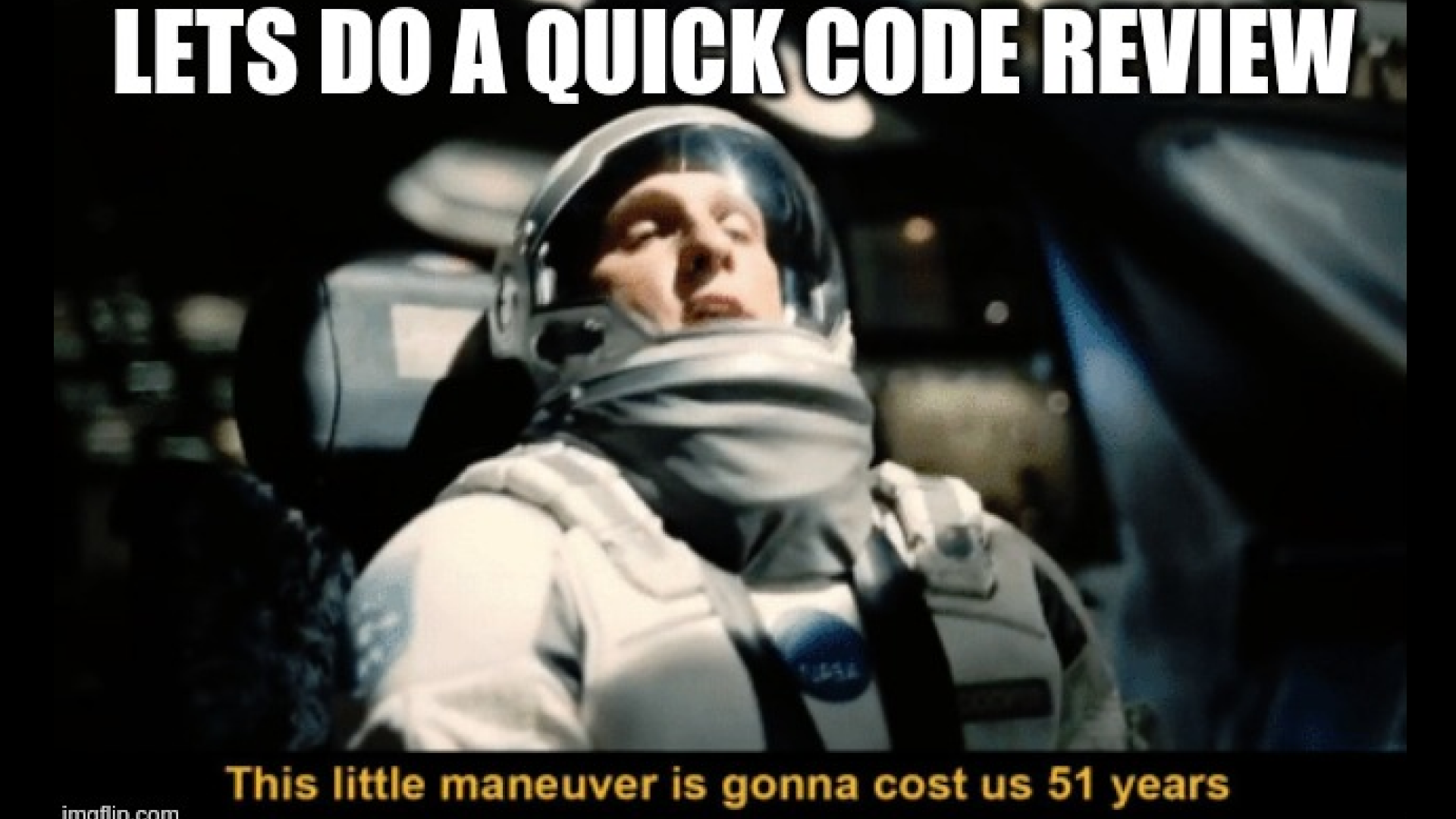
Problem:
Inconsistent code formatting across different contributors makes code reviews tedious and harms readability.
Solution:
Implement Prettier, Husky, and lint-staged to automatically format code with each git commit.
Benefits:
- No more "format-fighting" between contributors – consistency is king!
- Streamlined reviews – with automated styling, dive straight into the meat (or code) of the matter, sans the seasoning distractions.
Steps:
- Install dependencies
yarn add --dev --exact prettier
npm install husky@latest --save-dev
npx husky init
npm install --save-dev lint-staged
2. Define Formatting Rules with Prettier:
a. Create .prettierrc.js
for Prettier configurations:
module.exports = {
trailingComma: "es5", // Adds a trailing comma where valid in ES5
tabWidth: 2, // Sets the no of spaces per indentation-level
semi: false, // Opts out placing semicolons at end of lines
singleQuote: false, // Uses double quotes instead of single quotes
arrowParens: "always", // Always include parens for arrow functions
useTabs: false, // Uses spaces for indentation instead of tabs
printWidth: 120, // Wraps lines longer than 120 characters
proseWrap: "always", // Ensures consistent line breaks in md prose
}
b. Create .prettierignore
to exclude files and directories:
logs
node_modules
package-lock.json
yarn.lock
3. Configure Husky to Trigger lint-staged on Pre-commit:
a. Add lint-staged
configuration in package.json
:
"lint-staged": {
"*.{js,jsx,ts,tsx,json,css,scss,md}": [
"prettier --write",
"git add"
]
}
b. Configure Husky's pre-commit
hook:
Create (or modify) the .husky/pre-commit
file with the following content:
#!/bin/sh
. "$(dirname "$0")/_/husky.sh"
npx lint-staged
4. Setting Up Development and Start Scripts with Husky Permissions and Nodemon
scripts: {
"dev":"chmod +x .husky/* && next dev",
"start": "chmod +x .husky/* && nodemon --watch cloud ./index.js"
}